Your cart is currently empty!
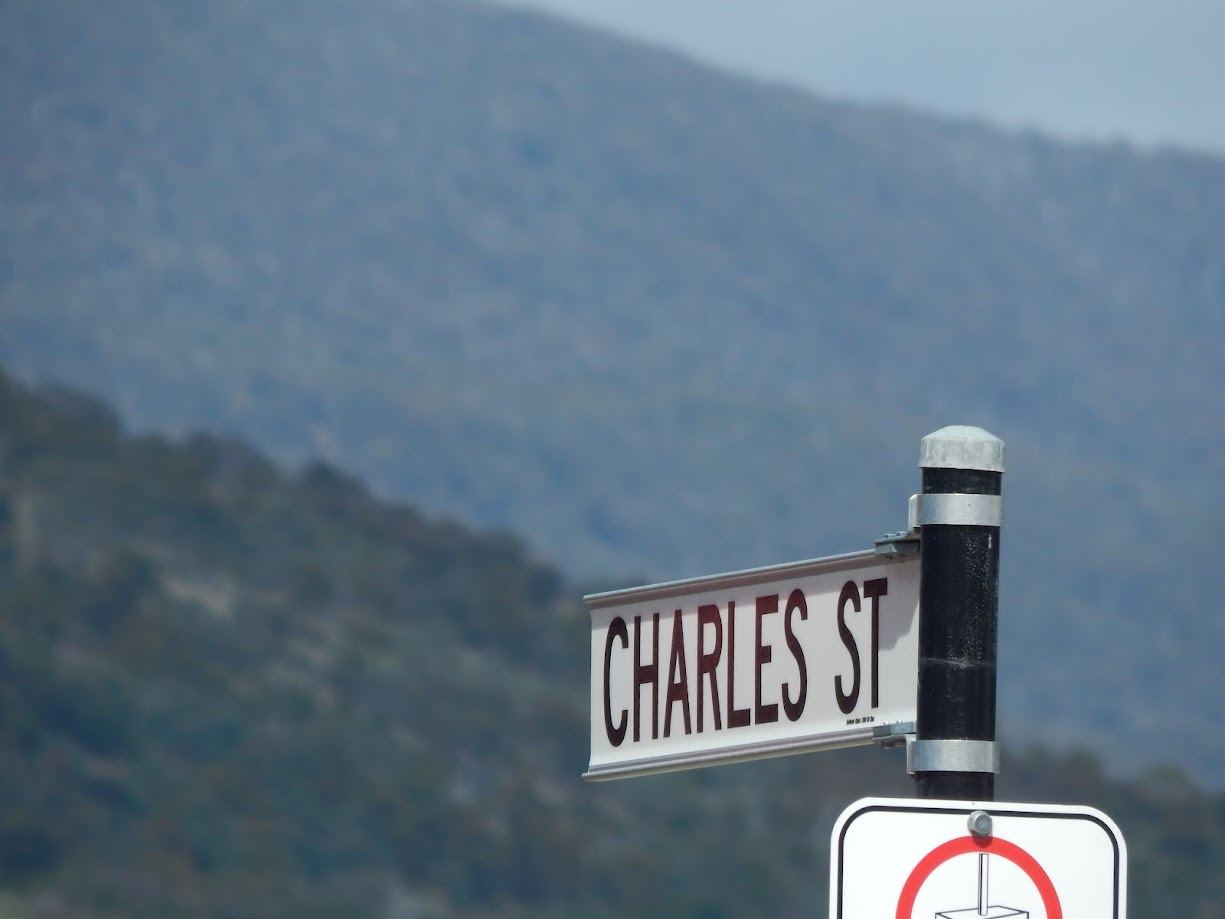
πΊοΈπποΈ Understanding Leaflet Map Events and Local Storage: How to Save and Load Map Bounds πΊοΈπποΈ
Leaflet is a powerful open-source JavaScript library that enables developers to create interactive maps with a range of tools and functionalities that can be customized to meet specific requirements. One of Leaflet’s essential features is the ability to save and load map bounds, which can be useful for various scenarios. In this blog post, we will explore how to use Leaflet’s map events and local storage to save and load map bounds.
Understanding the Code Snippet
The code snippet provided in the prompt shows three different functions that are used to save and load map bounds. Let’s go through each of them to understand how they work.
The first two functions, onMapZoomEnd() and onMapMoveEnd(), are event listeners that are triggered when the map is zoomed or moved. These functions get the current bounds of the map using the getBounds() method and save it in the local storage using the setItem() method.
The loadBounds() function is called when the page loads. It retrieves the saved bounds from the local storage using the getItem() method and checks if it is not null. If the saved bounds exist, it parses the JSON data and creates a new LatLngBounds object using the _northEast and _southWest properties. Then, it uses the flyToBounds() method to set the map’s bounds to the saved bounds. If the saved bounds do not exist, it calculates the bounds of the featureGroup and fits the map to those bounds.
Saving and Loading Map Bounds
To save the current map bounds, we need to add the onMapZoomEnd() and onMapMoveEnd() event listeners to the map object. Here’s an example:
map.on('zoomend', onMapZoomEnd);
map.on('moveend', onMapMoveEnd);
This will listen for the zoomend and moveend events and trigger the corresponding functions to save the map bounds.
To load the saved map bounds, we need to call the loadBounds() function when the page loads. Here’s an example:
window.addEventListener('load', loadBounds);
This will call the loadBounds() function when the page is loaded, and it will set the map bounds to the saved bounds if they exist.
Here is the full code:
onMapZoomEnd(e: ZoomAnimEvent | any) {
let bounds = this.map?.getBounds()
localStorage.setItem('bounds', JSON.stringify(bounds));
}
onMapMoveEnd(){
let bounds = this.map?.getBounds()
localStorage.setItem('bounds', JSON.stringify(bounds));
}
loadBounds() {
let savedBounds = localStorage.getItem('bounds') as string;
if (savedBounds !== null) {
let parsed = (JSON.parse(savedBounds) as any)
let bounds = L.latLngBounds(parsed._northEast,parsed._southWest)
this.map?.flyToBounds(bounds);
} else {
let b = this.featureGroup.getBounds();
if (this.featureGroup.getLayers().length > 0) {
this.map!.fitBounds(b);
}
}
}
You can test it out here: https://jcianci12.github.io/GeoJSON-Styler/
By using this method, you can easily save and load map bounds in Leaflet, providing a smoother experience for users who want to revisit their previous map views.
by
Leave a Reply