Your cart is currently empty!
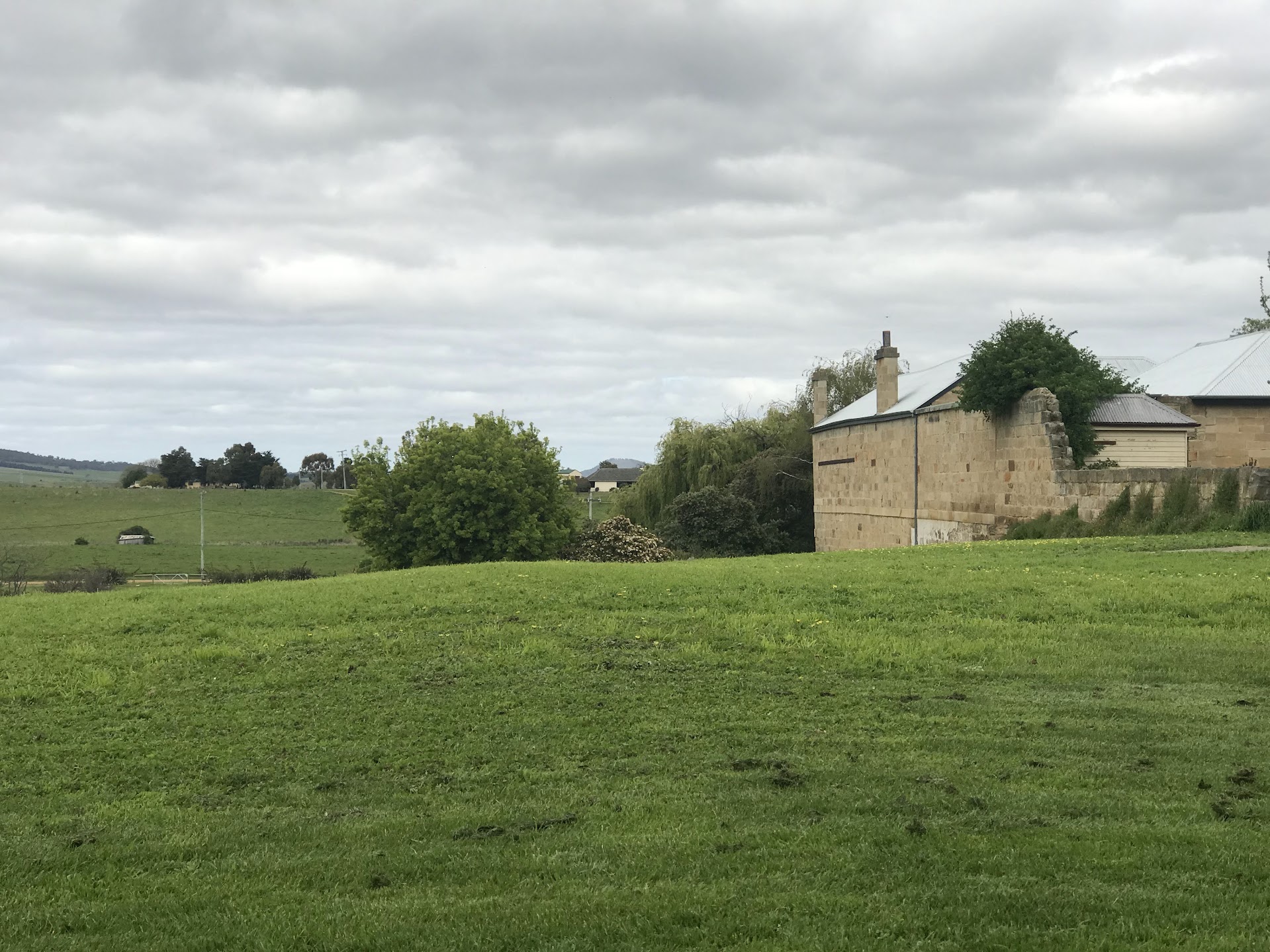
๐ How to Format and order dd-mm-yyyy Dates in jQuery DataTables ๐
If you’ve ever worked with DataTables, you may have encountered issues with how dates are displayed. By default, DataTables will display dates in a format that may not be easily readable or sortable. However, with a few simple tweaks, you can format your dates to display exactly how you want them.
First of all your going to need your column date data to be formated this way:
2023-06-30T00:00:00
In order to get this format in c# you can use this (in other languages it will vary):
YourDateYouWantToUse.Value.ToString("s", System.Globalization.CultureInfo.InvariantCulture)
Now we can format the date! One approach that has been found to be effective is by using the columnDefs
property of DataTables. Here’s how to use it to format your dates:
"columnDefs": [
{
"targets": 3,
"render": function (data, type, row) {
const date = new Date(data);
const day = ('0' + date.getDate()).slice(-2);
const month = ('0' + (date.getMonth() + 1)).slice(-2);
const year = date.getFullYear();
const formattedDate = `${day}-${month}-${year}`;
return "<span style='display:none'>"+data+"</span>"+ formattedDate;
}
}
],
Here, we’re targeting the 4th column (index 3) of our DataTable, which contains the dates we want to format. We’re using the render
function to format the dates, which takes in the data, type, and row as parameters.
First, we’re creating a new Date
object using the data
parameter. This assumes that data
is a valid date string that JavaScript can parse.
Next, we’re using some basic JavaScript date methods to extract the day, month, and year from the date object. We’re also adding leading zeros to the day and month using slice(-2)
so that they’re always two digits long.
Finally, we’re combining the day, month, and year into a string using template literals, and returning it as our formatted date. We’re also including a hidden span
element containing the original date string, in case we need it for further processing.
This method has been found to be effective for displaying and sorting dates in a readable format. Plus, it’s easily customizable if you need to change the format to something different.
๐ Note: When working with dates in JavaScript, be sure to pay attention to time zones and daylight saving time. Depending on your use case, you may need to adjust your date calculations accordingly.
In summary, formatting dates in DataTables is easily achievable using the columnDefs
property and a little bit of JavaScript. With this approach, you can display your dates exactly how you want them, making them more readable and sortable for your users.
Leave a Reply