Your cart is currently empty!
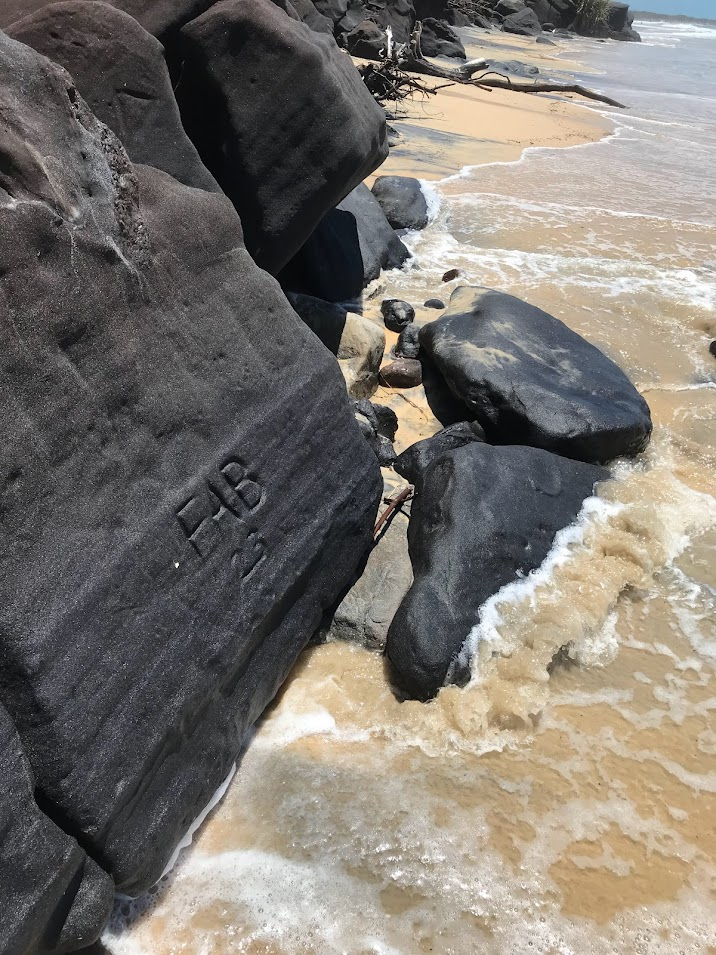
Working with Null dates in DateRange Picker.
๐ Hey there! I recently worked on implementing a date range picker using the Daterangepicker plugin in my web application. It was a bit of a challenge at first, but I learned a lot from the experience.
One of the main issues I encountered was how to handle null dates with moment.js library. I found that by setting the “autoUpdateInput” option to false, I was able to prevent the plugin from automatically updating the input field when a null value was selected.
$(function () {
$('.datepicker1').daterangepicker({
autoUpdateInput: false,
To handle null dates, I added the following code:
var startDateVal = start.isValid() ? start.format(formatstring) : null;
var endDateVal = end.isValid() ? end.format(formatstring) : null;
$(this.element).val(startDateVal + ' - ' + endDateVal);
This code checks whether the selected start and end dates are valid, and if so, formats them according to the date format string. If either date is null, it sets the corresponding variable to null. Finally, it updates the input field with the selected date range.
In addition to this fix, I also customized the Daterangepicker plugin with options such as “ranges” and “deploystartDate” and “deployendDate”. These options allowed me to define a set of predefined date ranges and to restrict the date range selection to a specific range.
To implement the code, I inserted it into my JavaScript file and called the Daterangepicker plugin on the appropriate HTML element with the class “datepicker1”. I also added a custom date validation method for the jQuery validation plugin, which used moment.js library to validate dates in the format “DD/MM/YYYY”.
<p>Deploy Date</p>
<input type="text" class="datepicker1 form-control" data-fieldtopopulateprefix="Deploy" name="StartDate" id="DateRangePicker" />
<input type="hidden" class="datepicker1" name="DeployEndDate" id="DeployEndDate" />
<input type="hidden" class="datepicker1" name="DeployStartDate" id="DeployStartDate" />
<p>Return Date</p>
<input type="text" class="datepicker1 form-control" data-fieldtopopulateprefix="Return" name="StartDate" id="DateRangePicker1" />
<input type="hidden" class="datepicker1" name="ReturnEndDate" id="ReturnEndDate" />
<input type="hidden" class="datepicker1" name="ReturnStartDate" id="ReturnStartDate" />
// Define format for datepicker
var formatstring = 'YYYY-MM-DDTHH:mm:ss';
// Declare variable to hold field prefix
var fieldPrefix;
// Initialize datepicker
$(function () {
$('.datepicker1').daterangepicker({
// Disable auto update of input field to handle null dates with moment
autoUpdateInput: false,
// Define date range options
ranges: {
'Today': [moment(), moment()],
'Yesterday': [moment().subtract(1, 'days'), moment().subtract(1, 'days')],
'Last 7 Days': [moment().subtract(6, 'days'), moment()],
'Last 30 Days': [moment().subtract(29, 'days'), moment()],
'This Month': [moment().startOf('month'), moment().endOf('month')],
'Last Month': [moment().subtract(1, 'month').startOf('month'), moment().subtract(1, 'month').endOf('month')],
'This Year': [moment().startOf('Year'), moment().endOf('Year')],
'Last 5 Years': [moment().startOf('Year').subtract(4, 'year'), moment().endOf('Year')],
'Empty': [null, null],
'All time': [moment("0001-01-01T00:00:00"), moment('9999-12-31T23:59:59.999Z')]
}
},
// Handle date range selection
function (start, end, label) {
// Get field prefix from data attribute
fieldPrefix = $(this.element).data('fieldtopopulateprefix');
// Format start and end dates for input fields
var startDateVal = start.isValid() ? start.format(formatstring) : null;
var endDateVal = end.isValid() ? end.format(formatstring) : null;
$('#' + fieldPrefix + 'StartDate').val(startDateVal);
$('#' + fieldPrefix + 'EndDate').val(endDateVal);
// Update input field with selected date range
$(this.element).val(startDateVal + ' - ' + endDateVal);
// Log selection and output start and end dates to console
console.log("A new date selection was made: " +
start.format(formatstring) +
' to ' +
end.format(formatstring), '#' + fieldPrefix + 'StartDate', '#' + fieldPrefix + 'EndDate'
);
console.log(start);
console.log(end);
// Reinitialize page
init();
})
// Reinitialize page on input or change to datepicker
.on("input change", function (e) {
console.log(e)
init();
});
// Handle cancel of datepicker
$('#DateRangePicker').on('cancel.daterangepicker', function (ev, picker) {
$(this).val('');
$('#' + fieldPrefix + 'StartDate').val('');
$('#' + fieldPrefix + 'EndDate').val('');
// Reinitialize page
//init();
});
});
// Add custom date validation using moment.js
$(function () {
$.validator.methods.date = function (value, element) {
return this.optional(element) || moment(value, "DD/MM/YYYY", true).isValid();
};
});
Overall, implementing a date range picker using the Daterangepicker plugin was a challenging but rewarding experience. By customizing the plugin and using moment.js library, I was able to create a user-friendly and reliable date selection tool for my web application.
๐ If you’re looking to implement a date range picker in your own web application, be sure to handle null dates with moment.js and customize the plugin to fit your specific needs.
Leave a Reply