Your cart is currently empty!
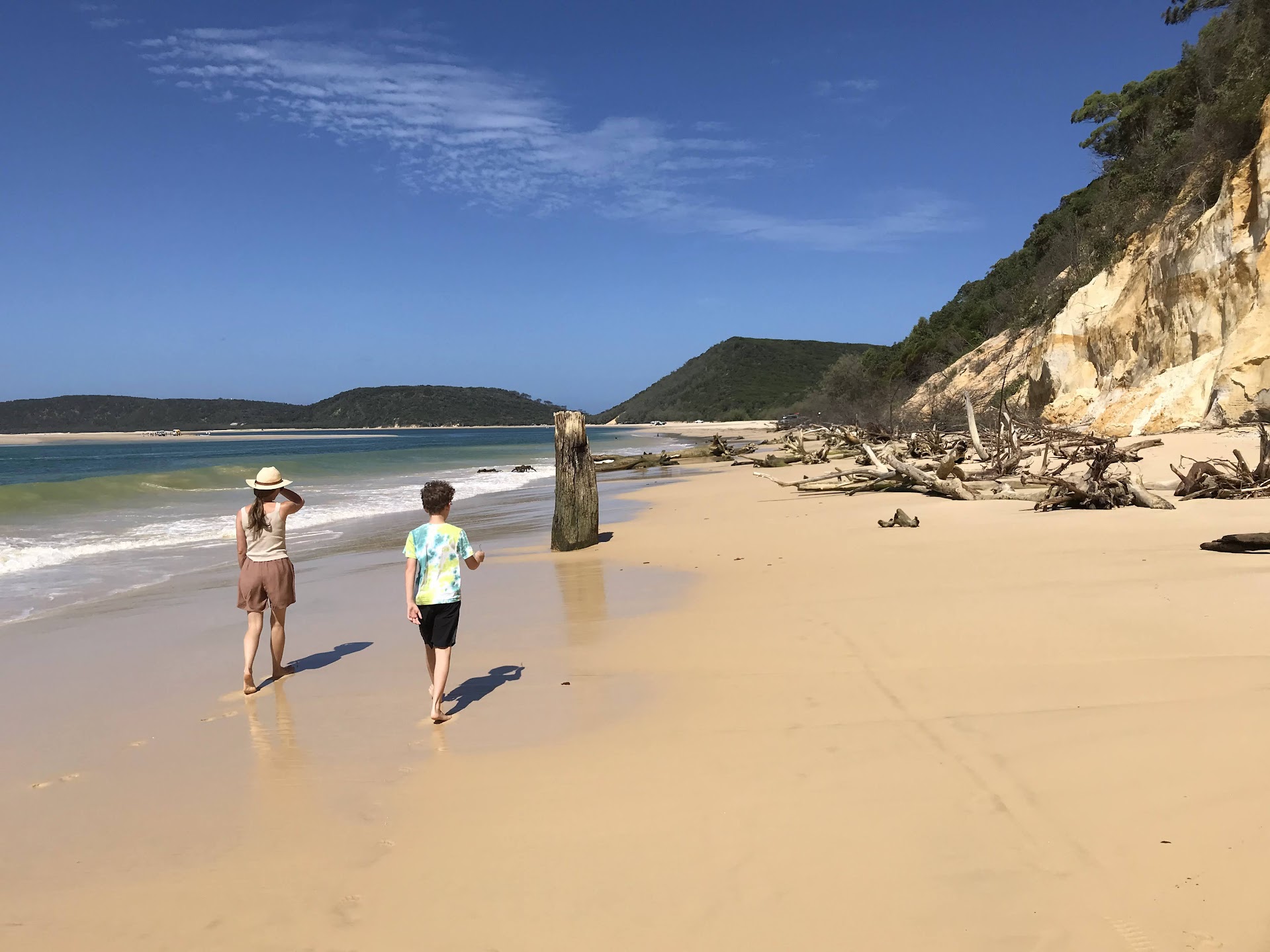
MVC 5 Dynamic redirect Uri for external OpenId authentication
If you are like me, you dont like having extra configuration in your web transforms.
Originally I was setting the redirect uri statically from the web config using
string redirectUri = System.Configuration.ConfigurationManager.AppSettings["RedirectUri"];
However, this means that we need a separate web config when we run locally vs when we run on the server. Also, the redirecturi is static. 😢
The change is quite simple, notice on line 33 we are tapping into the RedirectToIdentityProvider notification? Because this is fired at runtime, it means we also have the context. Because we have the context, we also have the URL. 😎
public void ConfigureAzureAuth(IAppBuilder app)
{
ServicePointManager.Expect100Continue = true;
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls
| SecurityProtocolType.Tls11
| SecurityProtocolType.Tls12
| SecurityProtocolType.Ssl3;
app.SetDefaultSignInAsAuthenticationType(CookieAuthenticationDefaults.AuthenticationType);
app.UseCookieAuthentication(new CookieAuthenticationOptions());
app.UseOpenIdConnectAuthentication(
new OpenIdConnectAuthenticationOptions
{
// Sets the ClientId, authority, RedirectUri as obtained from web.config
Caption = "Your site",
AuthenticationType = "Your site",
ClientId = clientId,
Authority = authority,
//MetadataAddress = "https://your auth url/.well-known/openid-configuration",
RedirectUri = redirectUri,
// PostLogoutRedirectUri is the page that users will be redirected to after sign-out. In this case, it is using the home page
PostLogoutRedirectUri = "/",
Scope = OpenIdConnectScope.OpenIdProfile,
// ResponseType is set to request the code id_token - which contains basic information about the signed-in user
ResponseType = OpenIdConnectResponseType.CodeIdToken,
// OpenIdConnectAuthenticationNotifications configures OWIN to send notification of failed authentications to OnAuthenticationFailed method
Notifications = new OpenIdConnectAuthenticationNotifications
{
AuthenticationFailed = OnAuthenticationFailed,
//We can tap into this notification to get the IOwinRequest object😎
RedirectToIdentityProvider = (o) =>
{
//here is where we set the redirect Uri based on the request we received.
o.ProtocolMessage.RedirectUri = DetermineRedirectUri(o.Request);
return Task.CompletedTask;
},
AuthorizationCodeReceived = (o) =>
{
o.TokenEndpointRequest.RedirectUri = DetermineRedirectUri(o.Request);
return Task.CompletedTask;
}
},
});
}
Based on some great feedback online, I kept my method to return a string from the request:
private string DetermineRedirectUri(IOwinRequest request)
{
return request.Scheme + System.Uri.SchemeDelimiter + request.Host + request.PathBase + "/Account/ExternalLoginCallback/";
}
by
Leave a Reply