Your cart is currently empty!
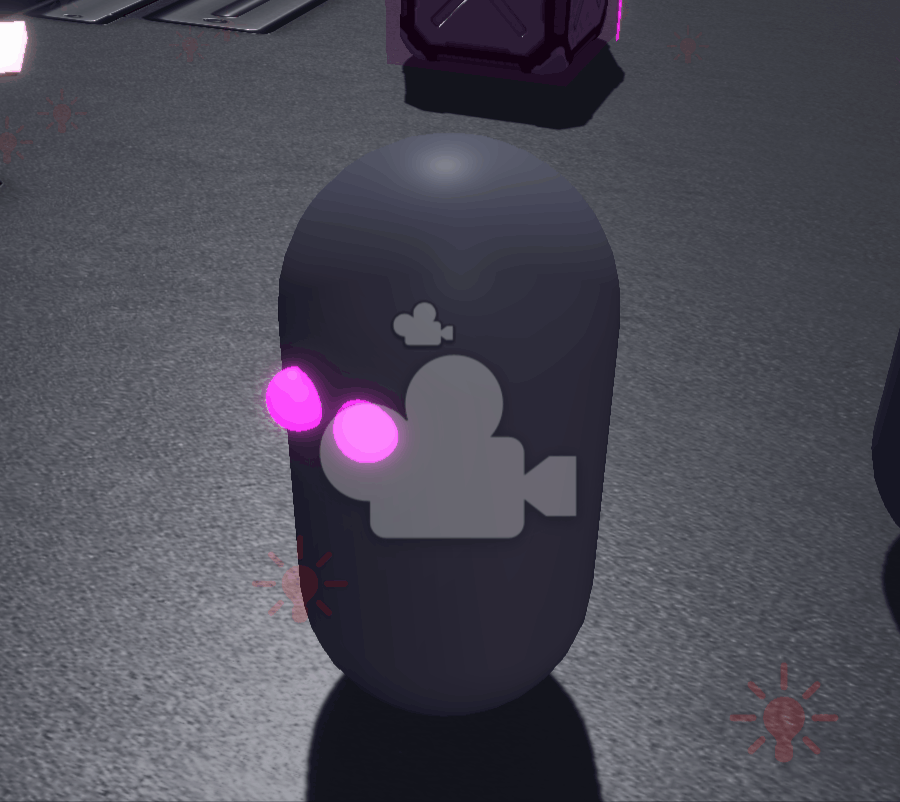
Limiting rotation in Unity easily, using relative direction of game objects

I wanted a way that would allow me to limit the gun rotation based on two game objects. That way different guns on the ship could have different limits so that the player couldn’t shot their own ship ๐
I wanted the limits to be visual, so that they are easy to visualize and adjust.
Overview of the Code
Let’s take a closer look at the code:
public GameObject limit1;
public GameObject limit2;
private Vector3 mindir;
private Vector3 maxdir;
public void FixedUpdate()
{
Debug.DrawRay(transform.position, transform.forward * 2, Color.red);
// Get the minimum and maximum directions for the gun rotation
mindir = (limit1.transform.position);
Debug.DrawLine(gun.transform.position, mindir, color:Color.green);
maxdir = (limit2.transform.position);
Debug.DrawLine(gun.transform.position, maxdir ,color:Color.magenta);
// Draw a line to show the movement direction of the gun
Debug.DrawLine(gun.transform.position + move.normalized, gun.transform.position, Color.yellow);
// Get the minimum and maximum angles for the gun rotation
float minangle = Vector3.SignedAngle(transform.forward, Vector3.Normalize(mindir - gun.transform.position), Vector3.up);
float maxangle = Vector3.SignedAngle(transform.forward, Vector3.Normalize(maxdir - gun.transform.position), Vector3.up);
// Get the current angle of the gun rotation
float currentangle = Vector3.SignedAngle(transform.forward, move, Vector3.up);
// Debug the angles for testing purposes
Debug.Log("minangle: " + minangle + " maxangle: " + maxangle + " move: " + currentangle);
// If the gun is being moved within the allowed range, rotate it
if (move != Vector3.zero && currentangle < maxangle && currentangle > minangle)
{
gun.transform.rotation = Quaternion.RotateTowards(gun.transform.rotation, Quaternion.LookRotation(move), Time.deltaTime * rotationSpeed);
}
}
This code is by no means optimized, but should give some good indications on how to solve your limiting rotation problems. ๐
Leave a Reply