Your cart is currently empty!
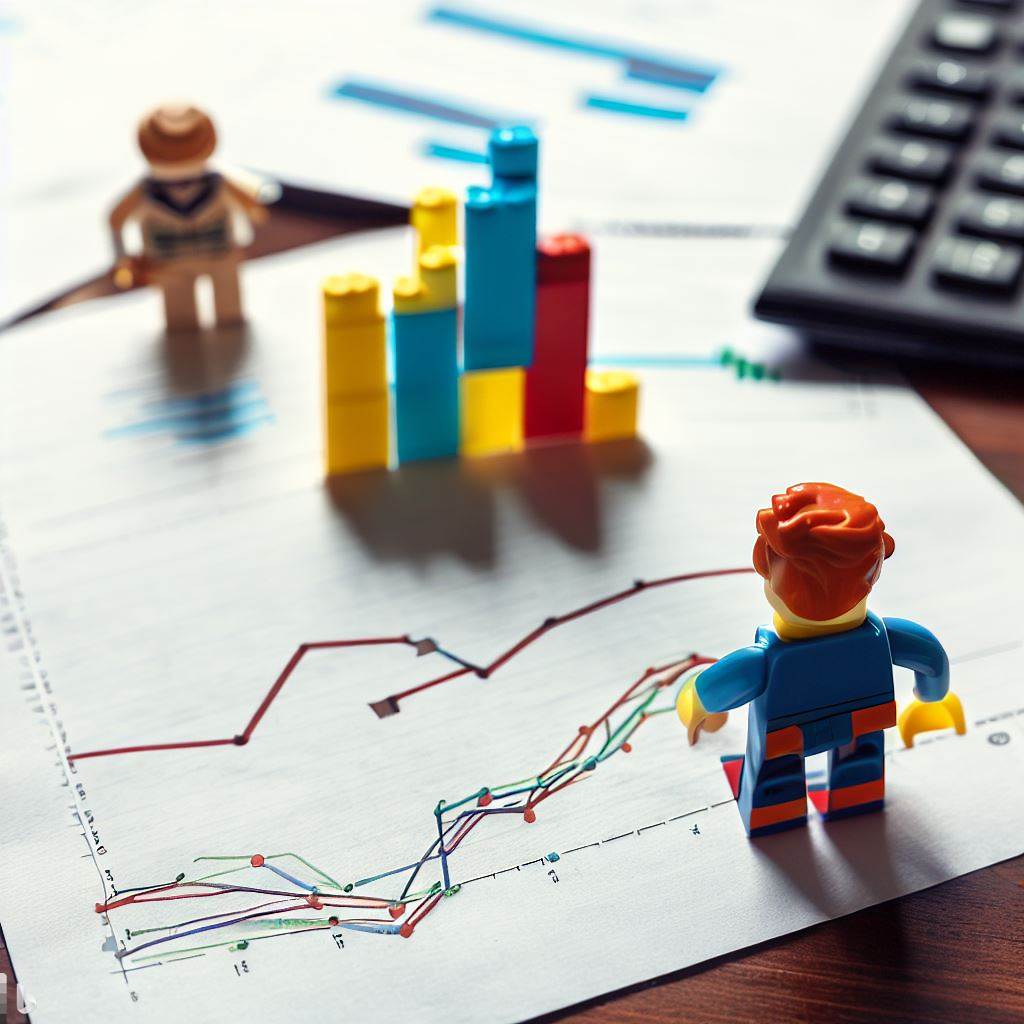
Debugging Adventures in Reinforcement Learning 🕹️🐞
Hello, fellow coders! Today, I want to share a recent debugging journey I embarked on while working with reinforcement learning (RL). It was a head-scratcher, but the solution brought that sweet “Aha!” moment we all know and love. So, buckle up and enjoy the ride! 🚀
The Setup 🏗️
I was working with a custom trading environment using OpenAI’s gym interface. My agent was using the Proximal Policy Optimization (PPO) algorithm from Stable Baselines 3 for training. The agent’s task was to navigate through OHLCV (Open, High, Low, Close, Volume) trading data.
The Problem 🧩
During training, I noticed something odd. The reset
method in my environment wasn’t being called at the start of each new episode as expected. This was puzzling because in RL, reset
is typically called automatically by the learning algorithm at the start of each new episode.
The Investigation 🔍
I started by checking if my environment correctly signaled when an episode was done. Everything seemed fine there. I also confirmed that PPO does support episodic environments, so that wasn’t the issue either.
To further investigate, I added a print statement in my environment’s reset
method:
def reset(self):
print("Reset method called")
...
This would print a message every time the reset
method was called. But alas, I didn’t see this message when I expected a new episode to start.
The Revelation 💡
After some more digging, I found the culprit. In my environment’s step
method, I was returning a variable named terminated
to indicate whether the current episode had ended. However, the convention in many RL environments and algorithms is to use a variable named done
for this purpose.
Here’s what my step
method looked like before:
def step(self, action):
...
terminated = (self.current_step == self.ret.shape[0]-1)
...
return observation, self.reward, terminated, truncated, info
And here it is after the fix:
def step(self, action):
...
done = (self.current_step == self.ret.shape[0]-1)
...
return observation, self.reward, done, truncated, info
The Conclusion 🎉
And voila! After this small change, everything worked as expected. The reset
method was being called at the start of each new episode.
This debugging adventure reminded me of how important it is to stick to naming conventions and understand the libraries we’re working with. It also reinforced how rewarding it can be to solve a tricky problem.
I hope you found this debugging story helpful or at least entertaining. Remember, every bug is a learning opportunity. Happy coding! 💻🎈
by
Leave a Reply