Your cart is currently empty!
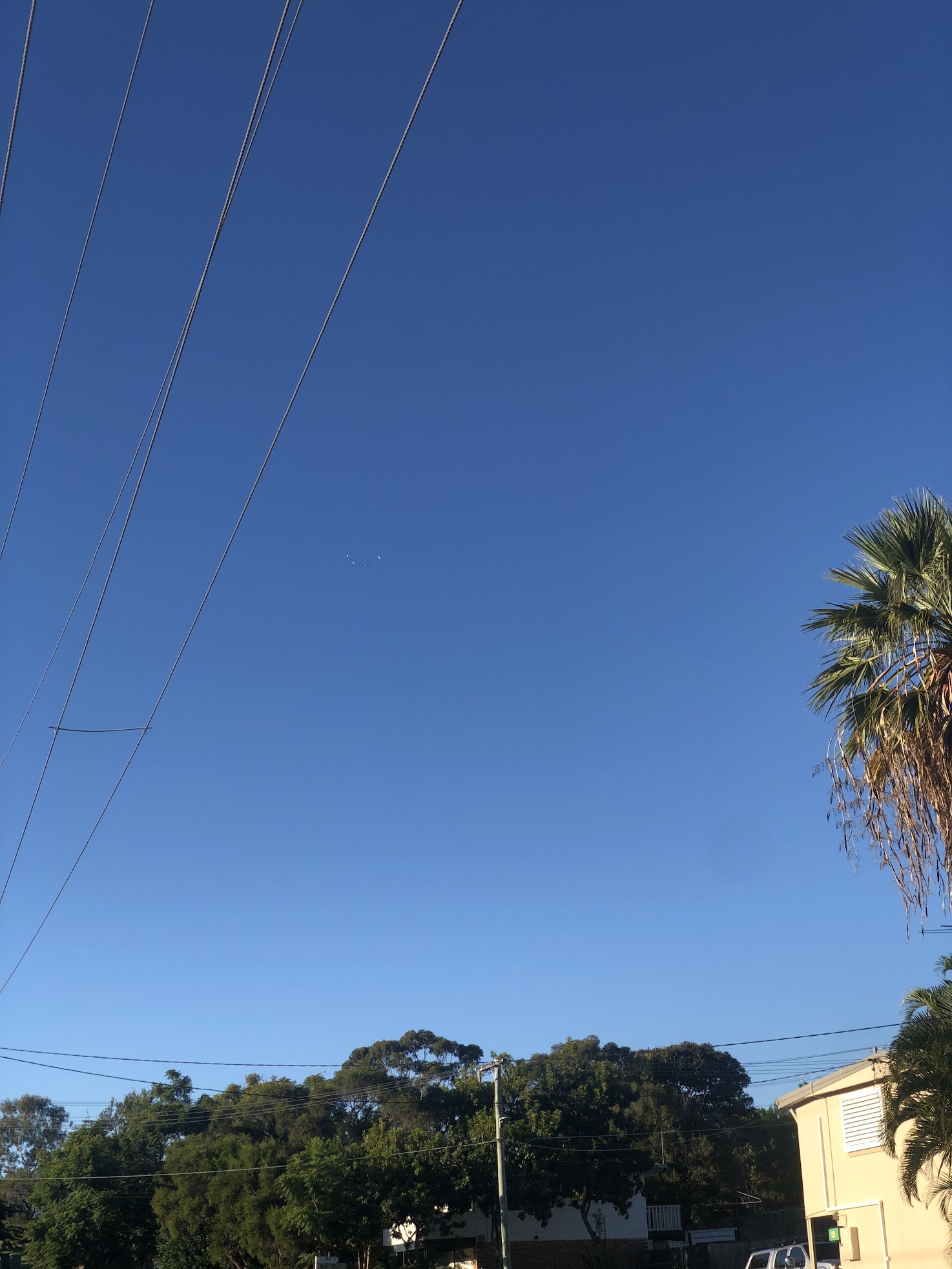
Converting from DOCX to PDF for thumbnails
I recently needed to show some thumbnails of docx files online. Here is what I came up with. Please note that this will not create a perfect thumbnail. In fact its quite basic. It allows the user to simply see that the file contains something. Nevertheless, I hope you find it helpful!
public ActionResult GetPDFFromDocx(string fileName, string OriginalName)
{
string docxFilePath = GetFileandPath(fileName);
string tempPdfFilePath = GetFileandPath(Guid.NewGuid().ToString("N") + ".pdf");
// Open the Word document
using (WordprocessingDocument wordDoc = WordprocessingDocument.Open(docxFilePath, false))
{
// Create a PDF document
using (PdfDocument pdfDoc = new PdfDocument())
{
// Add a new page to the PDF document
PdfPage page = pdfDoc.AddPage();
// Get the Word document body
Body body = wordDoc.MainDocumentPart.Document.Body;
// Render the Word document content to the PDF page
using (PdfSharp.Drawing.XGraphics gfx = PdfSharp.Drawing.XGraphics.FromPdfPage(page))
{
XTextFormatter tf = new XTextFormatter(gfx);
tf.DrawString(body.InnerText, new PdfSharp.Drawing.XFont("Arial", 12), PdfSharp.Drawing.XBrushes.Black,
new PdfSharp.Drawing.XRect(0, 0, page.Width, page.Height), PdfSharp.Drawing.XStringFormats.TopLeft);
}
// Save the PDF document to the specified file
pdfDoc.Save(tempPdfFilePath);
}
}
// Convert the docx file to pdf
var gf = GetFileContentResult(tempPdfFilePath, OriginalName);
// Delete the temporary files
System.IO.File.Delete(tempPdfFilePath);
return gf;
}
Here is the GetFileContentResult:
public FileContentResult GetFileContentResult(string path, string OriginalName)
{
if (!System.IO.File.Exists(path))
{
return null;
}
else
{
byte[] fileBytes = System.IO.File.ReadAllBytes(path);
return File(fileBytes, System.Net.Mime.MediaTypeNames.Application.Octet, OriginalName);
}
}
Leave a Reply