Your cart is currently empty!
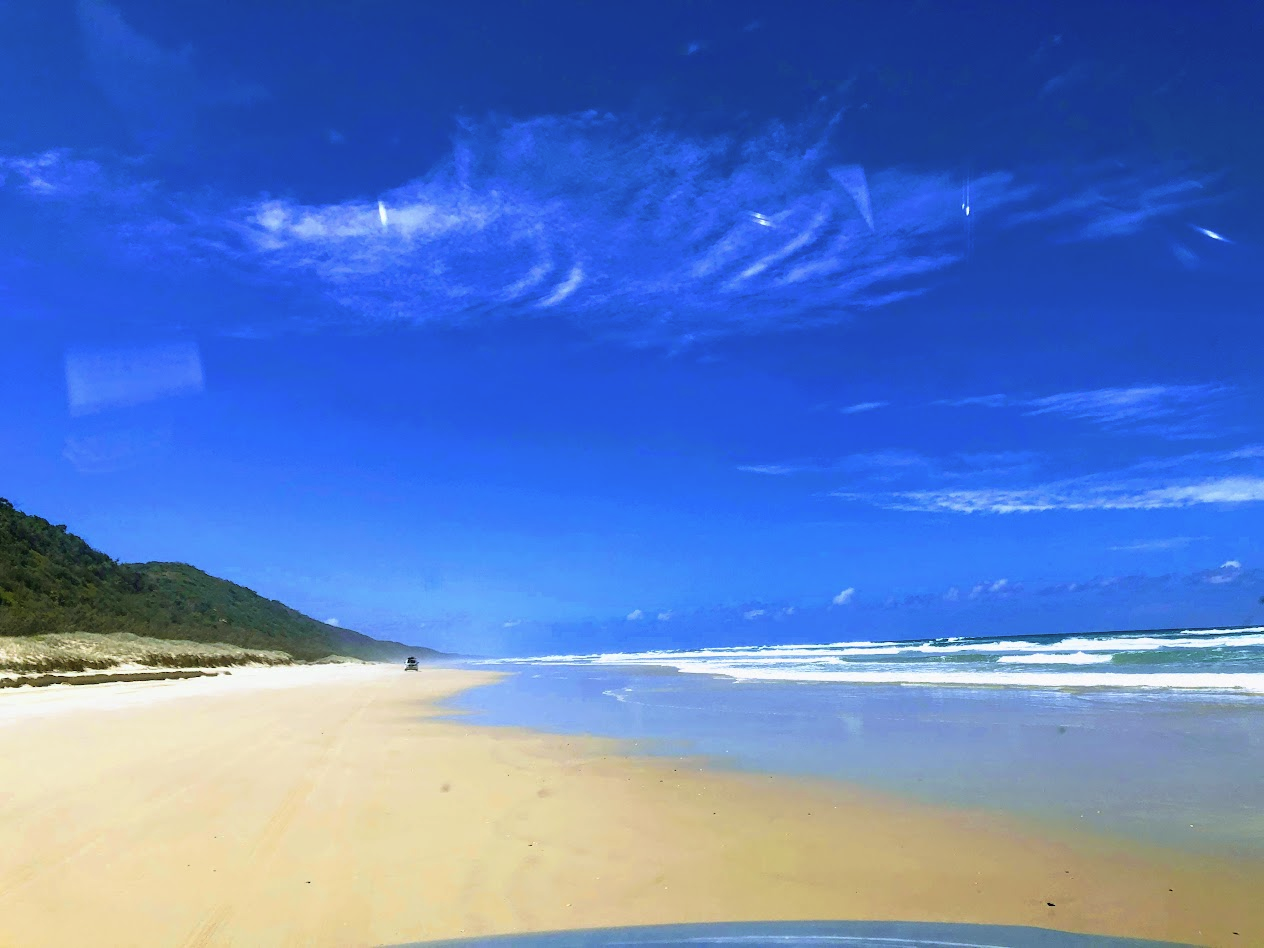
Angular JSON to CSV pipe
๐ Hey there! Are you working on an Angular app that needs to convert JSON data to CSV format? Look no further than the jsontocsv
pipe!
Here is the code:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'jsontocsv'
})
export class JsontocsvPipe implements PipeTransform {
// This function takes an object and returns a flattened version of it with dotted keys
// e.g. { foo: { bar: 'baz' } } becomes { 'foo.bar': 'baz' }
private flatten = (obj: any, prefix: string = ''): any => {
return Object.keys(obj).reduce((acc, k) => {
const pre = prefix.length ? prefix + '.' : '';
if (typeof obj[k] === 'object' && !Array.isArray(obj[k])) {
Object.assign(acc, this.flatten(obj[k], pre + k));
} else if (Array.isArray(obj[k])) {
obj[k].forEach((elem: any, i: number) => {
Object.assign(acc, this.flatten(elem, pre + k + `[${i}]`));
});
} else {
acc[pre + k] = obj[k];
}
return acc;
}, {});
};
// This function takes a JSON object as input and returns a CSV-formatted string
transform(jsonObj: any): string {
// If the input is an array, process each object in the array and return the CSV-formatted string
if (Array.isArray(jsonObj)) {
if (jsonObj.length === 0) {
return '';
}
// Get the headers for the CSV by flattening the first object in the array and joining the keys with commas
const headers = Object.keys(this.flatten(jsonObj[0])).join(',') + '\n';
// Convert each object in the array to a row in the CSV by flattening the object, getting the values, and joining them with commas
const rows = jsonObj
.map((row: any) => Object.values(this.flatten(row)).join(','))
.join('\n');
// Return the headers and rows joined together with a newline character
return headers + rows;
} else {
// If the input is an object (not an array), process the object and return the CSV-formatted string
// Get the headers for the CSV by flattening the object and joining the keys with commas
const headers = Object.keys(this.flatten(jsonObj)).join(',') + '\n';
// Get the values for the CSV by flattening the object, getting the values, and joining them with commas
const rows = Object.values(this.flatten(jsonObj))
.map((row: any) => Object.values(row).join(','))
.join('\n');
// Return the headers and rows joined together with a newline character
return headers + rows;
}
}
}
๐ง Let’s take a closer look at how this pipe works. First, it defines a private function called flatten
that takes an object and returns a flattened version of it with dotted keys. For example, { foo: { bar: 'baz' } }
becomes { 'foo.bar': 'baz' }
. This function is used later on to process the JSON data.
๐ The transform
function is the heart of the jsontocsv
pipe. It takes a JSON object as input and returns a CSV-formatted string. If the input is an array, each object in the array is processed and returned as a row in the CSV. If the input is an object (not an array), the object is processed and returned as a single row in the CSV.
๐ To get the headers for the CSV, the first object in the array (or the input object, if it’s not an array) is flattened using the flatten
function and the keys are joined with commas. To get the values for the CSV, each object in the array (or the input object) is flattened using the flatten
function, the values are retrieved, and they’re joined with commas.
๐ The result is a CSV-formatted string that can be used for a variety of purposes, such as exporting data to a spreadsheet program or generating reports.
You can check out our other article on converting CSV to JSON:
๐ So there you have it! The jsontocsv
pipe is a handy tool for converting JSON data to CSV format in your Angular app. Give it a try and see how it can streamline your data processing workflow. Happy coding!
You can check it out here:
by
Tags:
Comments
One response to “Angular JSON to CSV pipe”
-
[…] Angular JSON to CSV pipe […]
Leave a Reply