Your cart is currently empty!
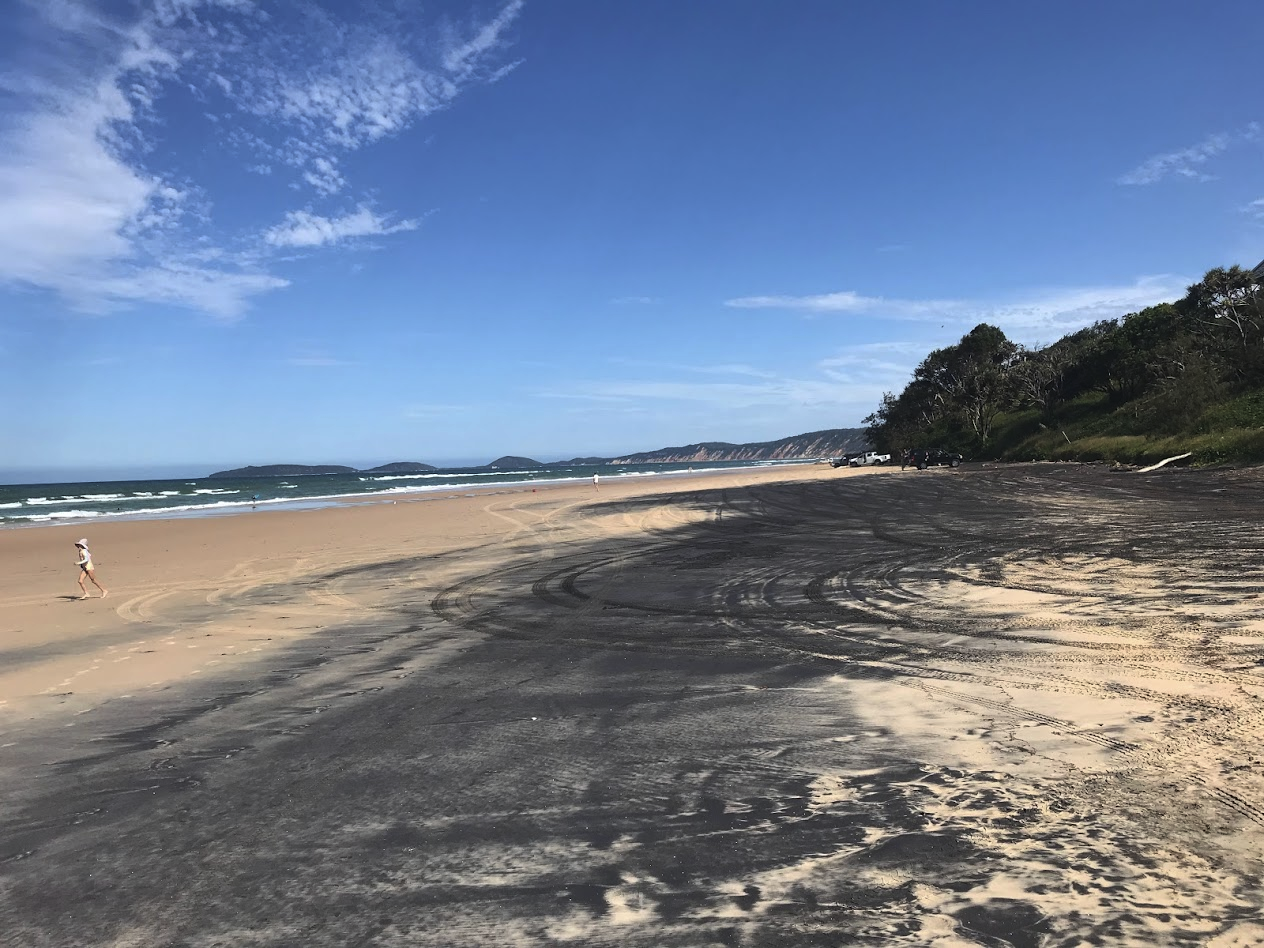
Handy angular CSV to JSON pipe
Today, we’re going to talk about a code snippet that can turn CSV data into JSON format using Angular. ๐ค๐ป
First things first, let’s take a look at the code:
import { Pipe, PipeTransform } from '@angular/core';
@Pipe({
name: 'csvtojson'
})
export class CsvtojsonPipe implements PipeTransform {
transform(value: string): string {
if (!value) return "";
const lines = value.split('\n');
const header = lines[0].split(',');
const result = [];
for (let i = 1; i < lines.length; i++) {
const obj = {};
const currentline = lines[i].split(',');
for (let j = 0; j < header.length; j++) {
obj[header[j]] = currentline[j] ? currentline[j].trim() : '';
}
result.push(obj);
}
return JSON.stringify(result);
}
}
This is a TypeScript class that defines an Angular pipe called CsvtojsonPipe
. A pipe is a way to transform data in Angular.
The transform
method of the CsvtojsonPipe
class is where the magic happens. It takes a string value (presumably a CSV-formatted string) as input and returns a JSON-formatted string.
Here’s what’s happening inside the transform
method:
- If the input value is falsy, return an empty string. This is just a safeguard in case the input value is undefined, null, or an empty string.
- Split the input value into an array of lines using the newline character (
\n
) as the delimiter. - Extract the header row of the CSV by splitting the first line using commas as the delimiter.
- Initialize an empty array called
result
. - Loop over each line of the CSV (starting from the second line) and create a new object for each line.
- Split the current line into an array using commas as the delimiter.
- Loop over each element in the header row and add a new key-value pair to the current object. The key is the header element and the value is the corresponding element in the current line. If the value is falsy, set it to an empty string.
- Add the current object to the
result
array. - After all lines have been processed, return the
result
array as a JSON-formatted string.
You can check out the implementation on a live site here:
https://jcianci12.github.io/converter/
Also here is the pipe to take the JSON and convert it to CSV
So, there you have it! This is a handy code snippet that can turn CSV data into JSON format using Angular. ๐ If you’re working with CSV data in your Angular project, you can use this pipe to quickly and easily transform it into a format that’s easier to work with. Happy coding! ๐๐จโ๐ป
You can check it out in action here:
by
Tags:
Comments
One response to “Handy angular CSV to JSON pipe”
-
[…] Handy angular CSV to JSON pipe CSV to JSON pipe […]
Leave a Reply