Your cart is currently empty!
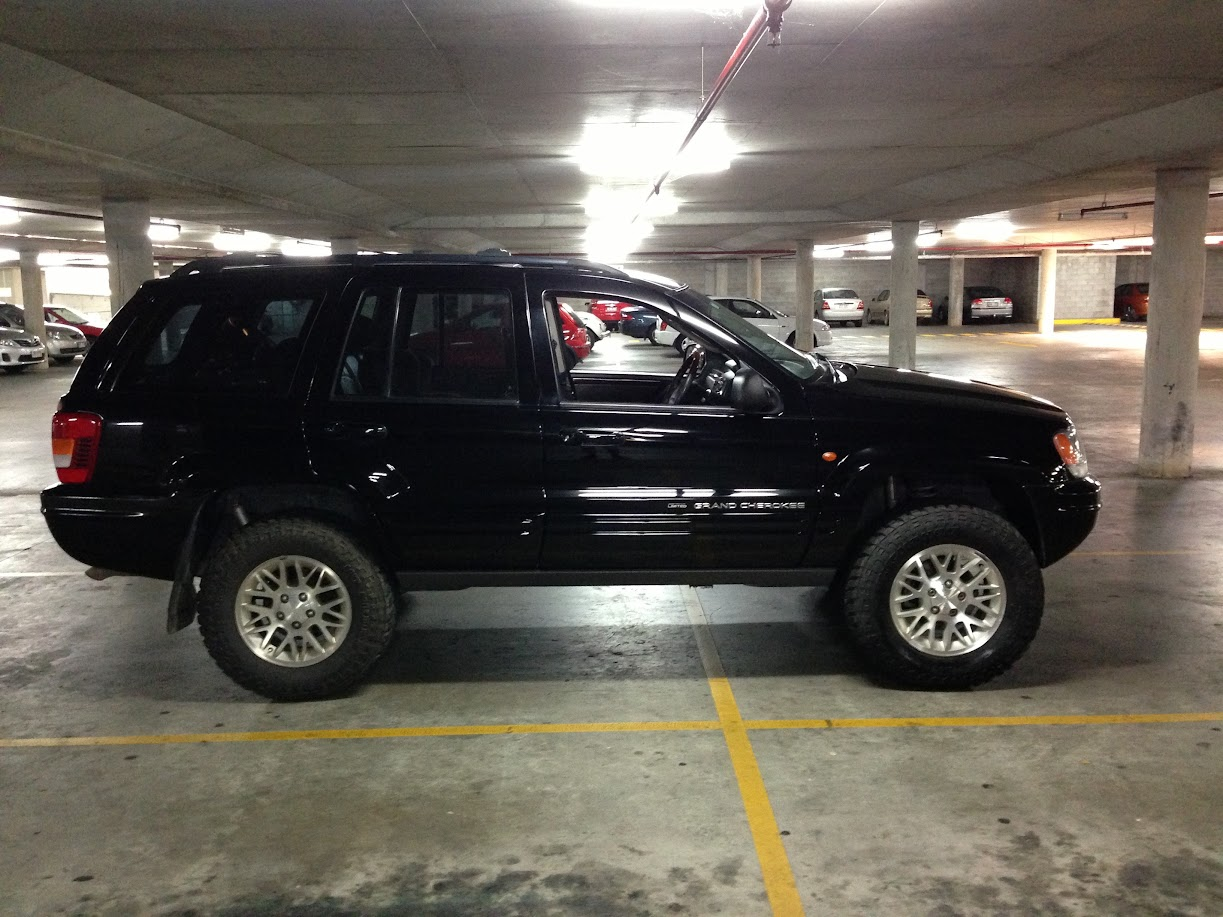
๐ป Angular Tip: How to Trigger a Pipe Update with Object Assignments
If you’re building an Angular app that uses pipes to transform data, you may run into a situation where you need to update an object in your component and have the pipe react to the change. But what if the pipe doesn’t update, even though you’ve assigned a new value to the object? ๐
Here’s an example to illustrate the issue:
// This one doesn't work
this.fcs.FeatureCollectionLayerObservable.subscribe((i) => {
this.featureCollectionLayer = i[this.featureCollectionIndex];
});
// This one does work
this.fcs.FeatureCollectionLayerObservable.subscribe((i) => {
this.featureCollectionLayer = new FeatureCollectionLayer(
i[this.featureCollectionIndex].features,
new terms(),
[],
{ GEOColumn: 'suburb', GEOJSON: 'qld_loca_2' }
);
});
In the first subscription, we assign a new value to this.featureCollectionLayer
by simply setting it equal to the value obtained from the observable. In the second subscription, we create a new object with the same data, but by using the new
keyword to create a new instance of the FeatureCollectionLayer
class.
But why does the first subscription fail to update the pipe, while the second one succeeds?
The answer lies in how Angular detects changes. When you assign a new value to an object property in your component, Angular only detects the change if the reference to the object changes. In the first subscription, we assign a new value to this.featureCollectionLayer
, but the reference to the object remains the same. Therefore, Angular doesn’t detect any change and doesn’t update the pipe.
In the second subscription, however, we create a new instance of the FeatureCollectionLayer
class, which means that the reference to the object is different from the previous one. This triggers Angular’s change detection mechanism, and the pipe updates accordingly.
So, what can you do if you need to update an object in your component and trigger a pipe update? The solution is to modify the object in place, rather than assigning a new value to it. For example:
this.fcs.FeatureCollectionLayerObservable.subscribe((i) => {
const featureCollection = i[this.featureCollectionIndex];
const featureCollectionLayer = this.featureCollectionLayer;
featureCollectionLayer.features = featureCollection.features;
this.cdr.markForCheck();
});
In this code, we first get the feature collection from the observable and store it in a local variable. We also get a reference to the existing FeatureCollectionLayer
object by accessing the this.featureCollectionLayer
property.
Then, we update the features
property of the existing object directly, instead of assigning a new value to the entire object. Finally, we call markForCheck()
on the ChangeDetectorRef
instance to manually trigger a change detection cycle, which will cause the pipe to update.
By modifying the object in place, Angular detects the change and triggers the pipe update, allowing you to display the updated data in your app.
In summary, when updating object properties in Angular, it’s important to keep in mind that Angular detects changes based on object references, not object values. By creating a new instance of an object, you can trigger a change detection cycle and update the pipe. But if you need to update an object in place, you can modify its properties directly and manually trigger a change detection cycle to achieve the same effect. ๐
by
Leave a Reply