Your cart is currently empty!
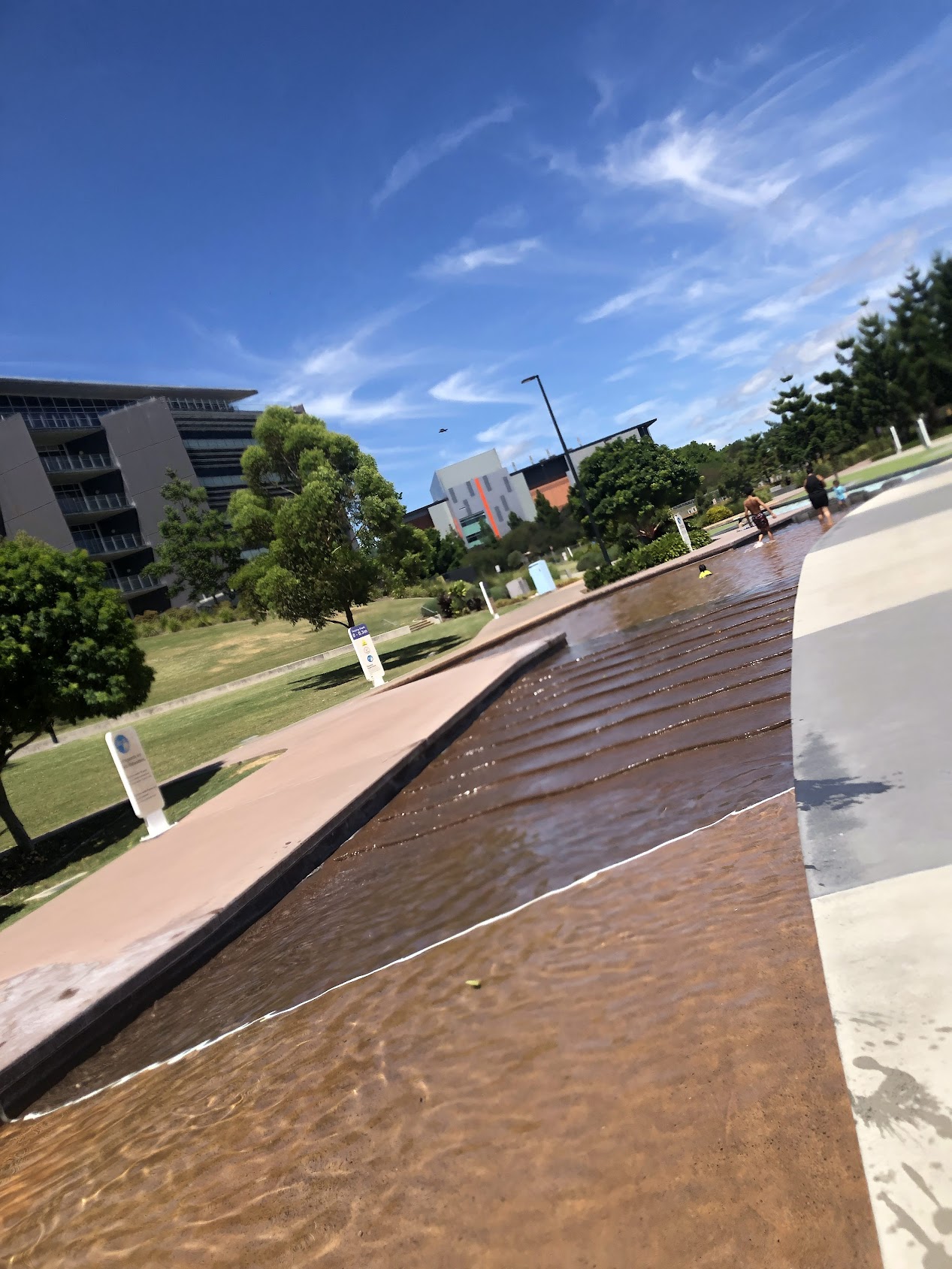
XPath Cheat Sheet 🎮
Hopefully you find these handy!
//
: Selects all elements that match the following path, regardless of their position in the document./<tag>
: Selects all elements with the specified tag name.[@attribute='value']
: Selects all elements that have a specified attribute with a specific value.//<tag>[@attribute='value']
: Selects all elements with the specified tag name that have a specified attribute with a specific value.contains(text(),'text')
: Selects all elements that contain the specified text.starts-with(@attribute,'value')
: Selects all elements that have a specified attribute with a value that starts with the specified text.normalize-space()
: Removes leading and trailing white space and collapses all other white space into a single space.parent::
or..
: Selects the parent element of the current element.following-sibling::
orfollowing::
: Selects the next sibling element(s) of the current element.preceding-sibling::
orpreceding::
: Selects the previous sibling element(s) of the current element.
Handy XPath operators
// Select by Contains Text
// Select element containing exact text (case-sensitive)
//*[contains(text(), 'exact text')]
// Select element containing text (case-insensitive)
//*[contains(translate(text(), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ', 'abcdefghijklmnopqrstuvwxyz'), 'text')]
// Select element containing text with leading/trailing spaces (case-insensitive)
//*[contains(translate(normalize-space(text()), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ', 'abcdefghijklmnopqrstuvwxyz'), 'text')]
// Select by Attribute Value Containing Text
// Select element with attribute value containing text (case-sensitive)
//*[@attributeName[contains(text(), 'exact text')]]
// Select element with attribute value containing text (case-insensitive)
//*[@attributeName[contains(translate(text(), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ', 'abcdefghijklmnopqrstuvwxyz'), 'text')]]
// Select element with attribute value containing text with leading/trailing spaces (case-insensitive)
//*[@attributeName[contains(translate(normalize-space(text()), 'ABCDEFGHIJKLMNOPQRSTUVWXYZ', 'abcdefghijklmnopqrstuvwxyz'), 'text')]]
Putting it all together🌼
Sure, here are a few examples of using XPath with Selenium in C#:
- Finding an element by ID:
IWebElement element = driver.FindElement(By.Id("element-id"));
- Finding an element by class name:
IWebElement element = driver.FindElement(By.ClassName("class-name"));
- Finding an element by name attribute:
IWebElement element = driver.FindElement(By.Name("name-attribute"));
- Finding an element by XPath:
IWebElement element = driver.FindElement(By.XPath("//tagname[@attribute='value']"));
- Finding an element by partial text:
IWebElement element = driver.FindElement(By.XPath("//tagname[contains(normalize-space(text()), 'partial text')]"));
- Finding an element by CSS selector:
IWebElement element = driver.FindElement(By.CssSelector("tagname[attribute='value']"));
Once you have found the element, you can interact with it using various methods such as Click()
, SendKeys()
, Clear()
, GetAttribute()
, GetCssValue()
, GetText()
, and more.
For example, to click on an element you would use:
element.Click();
And to get the text of an element you would use:
string text = element.Text;
Leave a Reply